Tutorial Functional Programming with Python
The world of programming continuously evolves, and one of the paradigms making waves is functional programming (FP). The author Christian Harms tutorial functional programming with python serves as a beacon for both novice and seasoned developers alike, illuminating the path towards cleaner, more maintainable code. By harnessing the power of FP principles in Python, programmers can embrace a new way of thinking about problems and solutions, leading to more robust software design.
In this comprehensive guide, we will journey through the intricacies of functional programming in Python, drawing inspiration from Christian Harms’ tutorials while expanding on them with practical insights and real-world examples. United Coders As we delve deeper, we will explore key concepts, tools, and libraries that elevate Python’s capabilities in functional programming.
Understanding Functional Programming: A Deeper Dive
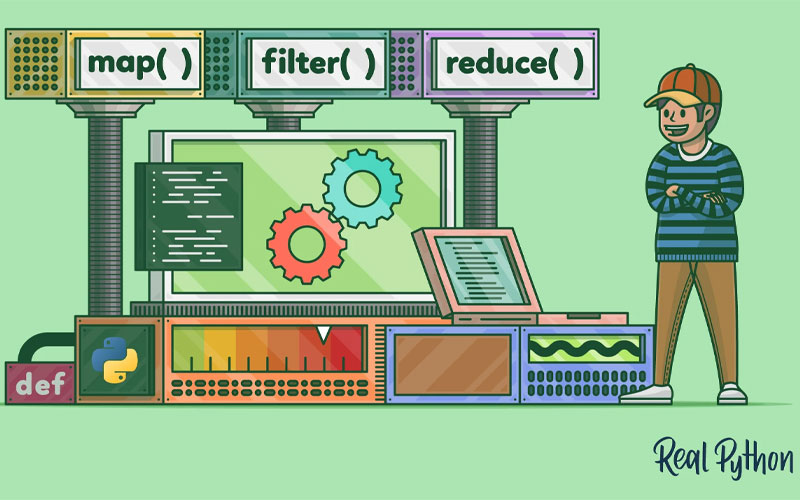
To fully appreciate the capabilities of functional programming within Python, it’s essential first to understand its foundational concepts. Each element plays a significant role in how FP enables developers to create elegant and efficient software solutions.
Pure Functions: The Cornerstone of FP
Pure functions are perhaps the most critical building block of functional programming. Defined as functions that yield the same output for the same input without side effects, they provide a level of predictability that can significantly enhance a codebase’s reliability.
The significance of pure functions extends beyond mere consistency. They promote isolation in the code, allowing developers to reason about their behavior independently of the broader application state. This encapsulation means that when testing or debugging, you can focus solely on the function in question, confident that external factors won’t influence its operation.
Moreover, when you rely on pure functions, your code becomes inherently easier to parallelize. Since pure functions don’t share state, they can be executed simultaneously without the risk of race conditions. This characteristic paves the way for writing concurrent applications, which is increasingly vital in today’s multi-core processing environments.
As an example, consider a simple function that computes the square of a number:
def square(x):
return x * x
This function fulfills all the criteria of a pure function. It always produces the same output for any given input, and it modifies no external state. The implications for testing and optimization are profound; you can easily set up unit tests for this function, guaranteeing its correctness without worrying about dependencies.
Immutability: Ensuring Data Integrity
Immutability is another pivotal tenet of functional programming. When data structures are immutable, they cannot be altered after creation. This immutability shields your program from unwanted side effects that can arise from unexpected changes to shared data, thus maintaining data integrity throughout the execution of your program.
In Python, several built-in data types exemplify immutability. Tuples and strings are two such examples, where once a tuple or string is created, it cannot be modified. This guarantees that functions relying on these data types will behave consistently over time.
To illustrate the power of immutability, consider a scenario where you’re working with a list of users. If you represent your user data using mutable lists, accidental modifications can lead to unwarranted bugs. Instead, by using tuples for your data representation, you create a safeguard against such issues:
users = (
('Alice', 30),
('Bob', 25),
)
In this case, the users’ information remains unchanged throughout the program, ensuring stability and predictability.
Embracing immutability not only enhances reliability but also aligns well with modern programming practices. In a world where data-driven applications are prevalent, understanding how to manage state effectively is paramount.
First-Class Functions: Empowering Functionality
One of the hallmarks of functional programming is the treatment of functions as first-class citizens. In Python, this means that functions can be passed around as values, returned from other functions, and assigned to variables just like any other data type.
This flexibility opens up a host of possibilities for abstraction and code organization. By treating functions as values, you can create higher-order functions – functions that either take other functions as arguments or return them as results. This ability allows developers to write highly modular and reusable code.
For instance, consider a higher-order function that accepts a mathematical operation and applies it to a list of numbers:
def apply_operation(operation, numbers):
return [operation(num) for num in numbers]
Using this approach, you could pass different operations (like squaring or doubling) to apply_operation
, providing exceptional versatility in how you process data.
Additionally, the use of first-class functions facilitates the implementation of callback mechanisms, event handling, and functional compositions, enriching the programmer’s toolkit for designing sophisticated systems.
By leveraging first-class functions, you elevate your coding practice, enabling you to think in terms of functionality rather than mere sequences of commands. This shift in perspective encourages you to create concise, expressive code that captures the essence of the problem at hand.
Higher-Order Functions: Abstracting Complexity
Higher-order functions (HOFs) take the concept of first-class functions a step further by allowing developers to abstract common patterns and simplify complex operations. These functions can accept other functions as parameters or return them as results, fostering code reusability and modularity.
Python comes equipped with built-in higher-order functions, such as map
, filter
, and reduce
. These functions enable you to apply transformations to collections of data concisely and fluently.
The map
function, for example, allows you to apply a transformation across an iterable. It can be a powerful ally when working with collections of data:
numbers = [1, 2, 3, 4, 5]
squared_numbers = list(map(lambda x: x * x, numbers))
In this snippet, map
composes a new list containing the squares of the original numbers. The beauty lies in its elegance; instead of writing verbose loops, you condense the logic into a single line.
Similarly, filter
lets you sift through collections based on criteria, returning only those elements that meet specified conditions. This simplifies data selection dramatically, enabling a declarative style of coding that highlights intent rather than implementation details:
even_numbers = list(filter(lambda x: x % 2 == 0, numbers))
Utilizing higher-order functions helps you separate concerns, promoting clearer code and reducing redundancy. As your codebase expands, HOFs empower you to implement complex logic without becoming mired in intricate constructions.
Tools and Libraries Enhancing Functional Programming in Python
Beyond core FP principles, Python boasts a plethora of features and libraries that facilitate functional programming practices. By leveraging these tools, you can accelerate your development process and create robust applications.
Lambda Expressions: Conciseness Meets Power
Lambda expressions are anonymous functions defined using a compact syntax. They allow you to create quick, throwaway functions without the need for formal definitions. This feature is particularly useful when working with higher-order functions.
Consider the following example using lambda
expressions with map
:
doubled_numbers = list(map(lambda x: x * 2, numbers))
Here, the lambda expression provides a concise way to define a function that doubles each item in the list. While it may appear succinct, care should be taken not to overuse lambdas, as overly complex expressions can become difficult to read and maintain.
Despite the potential pitfalls, leveraging lambda expressions judiciously can streamline your code by reducing verbosity. They offer a quick solution for scenarios where defining a full function would be cumbersome.
The functools Module: A Toolkit for Functional Programming
The functools
module is a treasure trove of utilities designed to enhance functional programming experiences in Python. From creating partial applications of functions to managing memoization, this module provides essential tools that every functional programmer should utilize.
One of the standout features of functools
is the partial
function. It allows you to fix certain arguments of a function, yielding a new function with reduced arity. This capability can lead to more readable code and decreased redundancy when working with multiple similar function calls:
from functools import partial
def multiply(x, y):
return x * y
double = partial(multiply, 2)
print(double(5))
# Output: 10
In this example, we create a new function called double
that multiplies its argument by 2. This illustrates how partial functions can encapsulate specific behavior, making your code cleaner and more expressive.
Another vital function in functools
is reduce
, which applies a binary function cumulatively to the items of a sequence. Although less frequently utilized than map
and filter
, reduce
can simplify certain operations on data structures:
from functools import reduce
total_sum = reduce(lambda x, y: x + y, numbers)
In this case, reduce
computes the sum of all numbers in the list. While some may prefer traditional looping constructs for readability, employing reduce
can lead to more elegant solutions in the right contexts.
List Comprehensions: Pythonic Data Handling
List comprehensions, a hallmark of Python’s expressiveness, offer a syntactically elegant way to create lists based on existing iterables. When used correctly, they can replace several lines of looping code with a single, coherent expression.
Consider a scenario where you want to extract the even numbers from a list:
even_numbers = [x for x in numbers if x % 2 == 0]
This example showcases how list comprehensions make your intention clear and direct. The benefits extend beyond brevity; they often result in better performance due to optimizations made internally by Python.
However, caution is warranted. Overly complex list comprehensions can hinder readability, especially when nested. Striking a balance between clarity and conciseness is crucial when utilizing this powerful feature.
Generator Expressions: Memory Efficiency in Action
While list comprehensions produce complete lists in memory, generator expressions create an iterator that generates items on-the-fly. This distinction makes generator expressions particularly valuable when dealing with large datasets.
A generator expression appears similar to a list comprehension but uses parentheses instead of brackets:
squared_numbers = (x * x for x in numbers)
for num in squared_numbers:
print(num)
This approach yields each squared number one at a time, conserving memory resources. In scenarios involving extensive calculations or streaming data, adopting generator expressions can lead to significant performance gains.
Generator expressions also align with the principles of functional programming, allowing you to create more declarative and composable code. By embracing this approach, you optimize your programs without sacrificing clarity.
Applying Functional Programming Principles: Practical Scenarios
Having established a strong theoretical foundation, let’s explore concrete applications of functional programming principles in solving real-world problems. By examining practical scenarios, we gain insight into how to leverage FP to craft efficient and elegant solutions.
Data Processing: A Functional Approach
Data processing tasks often require extracting, transforming, and analyzing information from various sources. By adopting a functional programming mindset, we can break down these tasks into smaller, manageable functions, enhancing both clarity and maintainability.
Imagine you have a list of dictionaries representing product information, and you want to extract the names of products priced above $100. By applying FP principles, you can achieve this with minimal overhead:
products = [
{'name': 'Laptop', 'price': 1200},
{'name': 'Keyboard', 'price': 50},
{'name': 'Monitor', 'price': 300},
{'name': 'Mouse', 'price': 20},
]
expensive_products = [product['name'] for product in products if product['price'] > 100]
print(expensive_products)
# Output: ['Laptop', 'Monitor']
In this example, we leverage list comprehensions to succinctly extract relevant information. The clarity of this approach stands in stark contrast to traditional looping constructs, underscoring the advantages of functional programming.
Furthermore, combining higher-order functions with filtering and mapping can amplify our data processing capabilities. For instance, imagine needing to retrieve discounted prices of products:
discounted_prices = list(map(lambda p: p['price'] * 0.9, filter(lambda p: p['price'] > 100, products)))
print(discounted_prices)
# Output: [1080.0, 270.0]
This demonstrates the power of chaining functional operations, resulting in clean, understandable code.
Text Analysis: Utilizing Functional Programming Techniques
Text analysis presents unique challenges that benefit immensely from functional programming principles. Word frequency counting offers an excellent illustration of how FP can simplify seemingly complex operations.
Suppose we want to count the frequency of words in a text file. By employing a combination of functional tools, we can achieve this efficiently:
from collections import Counter
def count_word_frequency(filename):
with open(filename, 'r') as file:
text = file.read().lower()
words = text.split()
word_counts = Counter(words)
return word_counts
word_frequencies = count_word_frequency('my_text_file.txt')
print(word_frequencies)
The use of Counter
from the collections
module illustrates the synergy of functional programming techniques and libraries. By organizing our logic into discrete functions, we enhance both the readability and maintainability of our code.
Furthermore, if you wish to extend this analysis, you could incorporate higher-order functions to manipulate or analyze the word frequencies further, showcasing the adaptability of FP principles.
Web Scraping: Structured and Streamlined Data Extraction
Web scraping often involves collecting data from various web pages and structuring that information for further analysis. Here, functional programming principles shine by enabling us to build streamlined and reusable components.
Consider a scenario where you want to scrape product data from a website. You can combine functional programming techniques to extract and structure that information efficiently:
import requests
from bs4 import BeautifulSoup
def scrape_product_data(url):
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
product_elements = soup.find_all('div', class_='product')
products = [extract_product_info(element) for element in product_elements]
return products
def extract_product_info(element):
return {
'name': element.find('h3').text,
'price': element.find('span', class_='price').text,
}
products = scrape_product_data('https://example.com/products')
print(products)
In this example, we’ve modularized our code into discrete functions, improving both readability and maintainability. Additionally, by leveraging list comprehensions, we efficiently gather product information while keeping our logic clean and intuitive.
Functional programming’s emphasis on composing small, focused functions lends itself beautifully to web scraping tasks. By structuring your code in this manner, you not only enhance clarity but also create reusable components that can adapt to varying scraping needs.
Conclusion: Embracing the Functional Way
The exploration of functional programming principles within Python reveals a powerful paradigm that can transform how we approach software development. With insights drawn from the author Christian Harms tutorial functional programming with python, we’ve highlighted key concepts like pure functions, immutability, and higher-order functions, demonstrating their relevance in practical scenarios.
By embracing functional programming principles, developers can unlock numerous benefits, from enhanced readability and maintainability to improved testability and reduced errors. Integrating these practices into your workflow can elevate your coding skills and empower you to create more resilient software.
As you continue your journey, remember that functional programming is not a rigid methodology but rather a flexible set of principles that can be incorporated gradually into your practices. Starting with small examples, you can progressively apply FP concepts to larger, more complex tasks, ultimately reaping the rewards of this dynamic paradigm.
In a rapidly evolving programming landscape, mastering functional programming in Python equips you with invaluable tools that foster creativity, efficiency, and excellence in software design.