Nico heid java persistence hibernate annotations is a powerful topic that combines the aspects of Java programming with the robust capabilities offered by Hibernate, an Object-Relational Mapping (ORM) framework. Understanding this subject can greatly enhance any developer’s ability to handle data in Java applications efficiently and effectively. In this article, we will explore various facets of Hibernate annotations, their usage, advantages, and how they contribute to Java persistence United Coders.
Introduction to Java Persistence API
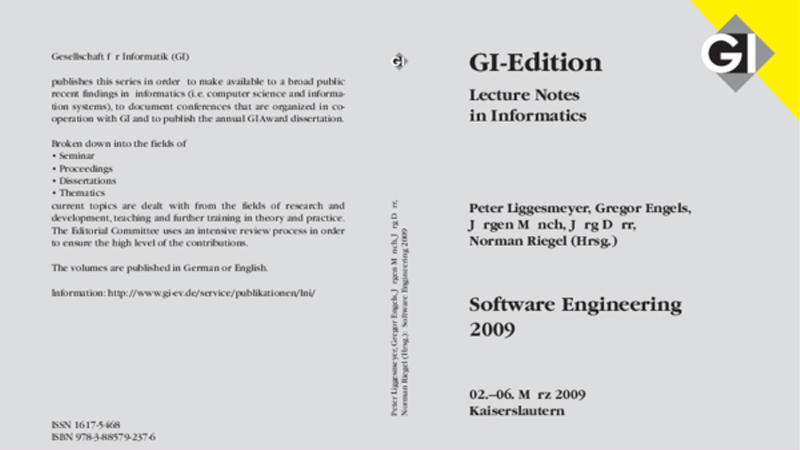
Java Persistence API (JPA) is a specification for accessing, persisting, and managing data between Java objects and relational databases. It serves as a bridge between the object-oriented domain model and the relational database model.
Overview of JPA
The Java Persistence API provides a set of guidelines and functionalities that allow developers to work with persistent data. The key advantage of using JPA lies in its ability to disconnect the application logic from the underlying database. This means that developers can focus on business logic without being bogged down by the complexities of SQL queries or database connections.
JPA standardizes how Java applications interact with databases, ensuring that developers can write code once and use it across different types of relational databases. With JPA, you can define entity classes, create relationships between these entities, and perform CRUD operations seamlessly.
Benefits of Using JPA
Utilizing JPA offers numerous benefits, including:
- Decoupling of Domain and Data Models: JPA allows for a clear separation between domain logic and data access logic. Developers can design their domain models without worrying about database structure.
- Portability: Applications built with JPA can be run on any platform that supports the JPA specification, making it easier to switch databases if necessary.
- Reduced Boilerplate Code: JPA’s annotations eliminate the need for verbose boilerplate code typically associated with JDBC.
- Improved Performance: Through features like caching and lazy loading, JPA can optimize performance, reducing database access times significantly.
Common Use Cases for JPA
Common scenarios where JPA shines include:
- Enterprise applications where complex data models are involved.
- Web applications requiring dynamic content retrieval from relational databases.
- Any project needing a rapid development cycle with constantly changing data requirements.
With a solid understanding of JPA, we can now transition into the nuances of Hibernate—an implementation of the JPA.
Deep Dive into Hibernate Annotations
Hibernate is more than just a JPA implementation; it is equipped with advanced features that facilitate working with Java persistence. One of the critical components of Hibernate is its use of annotations, which simplifies the mapping between Java classes and database tables.
Importance of Annotations in Hibernate
Annotations serve as metadata in Hibernate, providing information about how Java objects should be persisted in a relational database. They eliminate the need for XML configuration files, allowing for cleaner and more maintainable code.
Annotations are intuitive and closely resemble the structure of the Java code itself, making it easier for developers to understand the mappings at a glance. This leads to improved readability and maintainability.
Key Hibernate Annotations
Several annotations form the backbone of Hibernate’s functionality. Here are some of the most essential ones:
@Entity
The @Entity
annotation indicates that a class is an entity and is mapped to a database table. When using this annotation, Hibernate recognizes the class as a persistent entity and generates the necessary SQL statements to manage it in the database.
The @Entity
annotation needs to be placed above the class definition and is often accompanied by other annotations to fine-tune its behavior.
@Table
The @Table
annotation specifies the database table that corresponds to the entity. If not explicitly defined, Hibernate assumes the table name is the same as the entity class name.
Using the @Table
annotation allows you to customize the table name, define unique constraints, and set schema details.
@Id
The @Id
annotation designates the primary key of the entity. Every entity must have a primary key to uniquely identify its instances within the database.
This annotation can be combined with others, such as @GeneratedValue
, which controls how the primary key is generated (auto-increment, sequence, etc.).
Advanced Annotation Features
Beyond the basic annotations, Hibernate also supports advanced features through additional annotations, enhancing the power of JPA.
@OneToMany and @ManyToOne
These annotations establish relationships between entities. For example, @OneToMany
indicates that one instance of an entity relates to multiple instances of another entity.
Properly utilizing these relationship annotations helps maintain data integrity and reflects the real-world associations among objects.
@ManyToMany
When an association requires both sides to reference each other, the @ManyToMany
annotation comes into play. This is particularly useful for implementing many-to-many relationships, such as students enrolled in multiple courses.
Establishing these relationships through annotations reduces complexity, promoting clarity within the codebase.
@Transient
The @Transient
annotation marks fields in an entity that should not be persisted to the database. This is especially valuable when there is derived data or temporary fields that do not require storage, helping maintain clean data models.
By using the @Transient
annotation wisely, you can avoid unnecessary clutter in your database and improve overall performance.
Practical Examples of Hibernate Annotations
Putting theory into practice is crucial for grasping Hibernate annotations. In this section, we’ll explore practical implementations of some of the most commonly used Hibernate annotations.
Building a Simple Entity Class
Let’s create a simple entity class called User
. This class will have basic attributes like id
, name
, and email
.
import javax.persistence.*;
@Entity
@Table(name = "users")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "name", nullable = false)
private String name;
@Column(name = "email", unique = true, nullable = false)
private String email;
// Getters and Setters
}
In this example, the @Entity
annotation identifies the class as a Hibernate entity, while the @Table
annotation specifies the corresponding database table. The @Id
and @GeneratedValue
annotations designate the primary key and its generation strategy, respectively. Finally, @Column
allows customization of the column definitions.
Creating Relationships Between Entities
Now let’s introduce relationships by adding another entity, Order
, which represents user orders.
import javax.persistence.*;
import java.util.Set;
@Entity
@Table(name = "orders")
public class Order {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@ManyToOne
@JoinColumn(name = "user_id", nullable = false)
private User user;
@Column(name = "product", nullable = false)
private String product;
// Getters and Setters
}
Here, we use the @ManyToOne
annotation to denote that multiple orders can be linked to a single user. The @JoinColumn
annotation specifies the foreign key column in the orders
table.
Querying with Hibernate Annotations
Hibernate makes it easy to query data using HQL (Hibernate Query Language). You can leverage the annotations in your entity classes while constructing queries.
For instance, if we want to retrieve all orders for a specific user, we can easily perform the following query:
List orders = session.createQuery("FROM Order o WHERE o.user.id = :userId", Order.class)
.setParameter("userId", userId)
.getResultList();
This query utilizes Hibernate’s capabilities to translate the HQL statement into SQL while respecting the entity relationships defined through annotations.
FAQs
What is the role of Hibernate in Java Persistence?
Hibernate acts as an implementation of Java Persistence API (JPA), facilitating the mapping of Java classes to database tables and providing capabilities for CRUD operations.
Can Hibernate annotations replace XML configurations?
Yes, Hibernate supports the use of annotations instead of XML configuration files, allowing for a cleaner and more maintainable codebase.
How does the @GeneratedValue annotation work?
The @GeneratedValue
annotation defines strategies for generating primary key values automatically, such as auto-increment or sequence generation.
What is the difference between @OneToMany and @ManyToOne?
@OneToMany
establishes a one-to-many relationship where one instance of an entity relates to multiple instances of another entity, while @ManyToOne
indicates that multiple instances of an entity can relate to one instance of another entity.
What happens if I do not specify the @Id annotation?
Without the @Id
annotation, Hibernate will not recognize the entity as persistent, rendering it incapable of being saved to or retrieved from the database.
Conclusion
Nico heid java persistence hibernate annotations encapsulate a vast and powerful framework for handling data management in Java applications. By understanding the concepts of JPA and the application of Hibernate annotations, developers can build sophisticated enterprise applications that are efficient, maintainable, and scalable.
The flexibility offered by annotations simplifies the development process, allowing developers to spend less time on boilerplate code and more time focusing on core business logic. As technology continues to evolve, mastering concepts such as Hibernate annotations will remain integral to successful Java application development.